- Basic Concepts of Dictionaries in Python
- Example of a Dictionary:
- Accessing Values:
- Modifying a Dictionary:
- Deleting Key-Value Pairs:
- Advanced Dictionary Features – Python Dictionaries
- Dictionary Methods and Functions
- 1. .get() – Safely Access Values
- 2. .keys() – Retrieve All Keys
- 3. .values() – Retrieve All Values
- 4. .items() – Retrieve All Key-Value Pairs
- 5. .update() – Merge or Update Dictionaries
- 6. .pop() – Remove a Key-Value Pair and Return Its Value
- 7. .popitem() – Remove and Return the Last Key-Value Pair
- 8. .clear() – Remove All Items
- 9. .copy() – Create a Shallow Copy
- 10. .setdefault() – Get Value or Insert Default
- Other Useful Functions with Dictionaries
- Conclusion
Basic Concepts of Dictionaries in Python
Dictionaries in Python are a data structure that stores information in key-value pairs. They are similar to real-world dictionaries, where each word (key) maps to a specific definition (value). Python dictionaries are:
- Unordered: The elements in a dictionary are not stored in a specific sequence (though in Python 3.7 and later, dictionaries maintain insertion order).
- Mutable: You can change or update a dictionary after it’s created.
- Indexed by keys: Each key is unique and immutable (e.g., strings, numbers, or tuples), while the values can be of any data type (e.g., strings, lists, other dictionaries).
Key Characteristics:
- Keys must be unique: No two keys can be the same, but values can be repeated.
- Values can be any type: Strings, numbers, lists, or even other dictionaries.
- Keys are immutable: You can use types like strings or numbers as keys, but mutable types like lists cannot be used as dictionary keys.
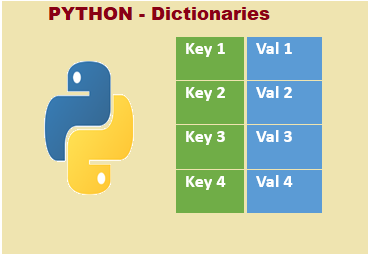
Example of a Dictionary:
my_dict = {
'name': 'Alice',
'age': 25,
'city': 'New York'
}
In this example:
- ‘name’, ‘age’, and ‘city’ are keys.
- ‘Alice’, 25, and ‘New York’ are the corresponding values.
Accessing Values:
To retrieve a value, use its key:
print(my_dict['name']) # Output: Alice
Modifying a Dictionary:
You can add or update key-value pairs:
my_dict['age'] = 26 # Updates the value for 'age'
my_dict['job'] = 'Engineer' # Adds a new key-value pair
Deleting Key-Value Pairs:
You can remove an entry using the del statement:
del my_dict['city'] # Removes the key 'city' and its value
This foundational understanding of Python dictionaries will help you organize data efficiently using key-value mapping.
Let’s dive deeper into some more advanced features of Python dictionaries, such as dictionary comprehension, default dictionaries, and merging dictionaries. These advanced techniques will help you write cleaner, more efficient code when working with dictionaries.
Advanced Dictionary Features – Python Dictionaries
1. Dictionary Comprehension
Dictionary comprehension is a concise way to create dictionaries, much like list comprehension but for key-value pairs. It allows you to generate a dictionary in a single line of code, making it more readable and efficient.
Example:
Let’s create a dictionary where the keys are numbers and the values are the squares of those numbers.
# Using dictionary comprehension to create a dictionary of squares
squares = {num: num**2 for num in range(1, 6)}
# Printing the resulting dictionary
print(squares)
Output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
Step-by-Step Explanation:
- Comprehension Syntax:
- The syntax {key: value for item in iterable} is used to create a dictionary.
- Here, num is both the key and the base for squaring, and num**2 represents the value.
- The range(1, 6) creates an iterable from 1 to 5.
- Dictionary Output:
- The result is a dictionary with numbers as keys and their squares as values.
2. Using defaultdict from the collections Module
A defaultdict is a subclass of Python’s built-in dictionary that provides a default value for a key that does not exist. This eliminates the need for explicit error handling or checking whether a key is present in the dictionary before using it.
Example:
Let’s create a defaultdict where the default value for missing keys is an empty list.
Code:
from collections import defaultdict
# Creating a defaultdict with list as the default data type
default_dict = defaultdict(list)
# Adding values to the dictionary
default_dict['fruits'].append('apple')
default_dict['fruits'].append('banana')
default_dict['vegetables'].append('carrot')
# Accessing a non-existent key
print('Fruits:', default_dict['fruits'])
print('Vegetables:', default_dict['vegetables'])
print('Dairy:', default_dict['dairy']) # No error, will return an empty list
Output:
Fruits: ['apple', 'banana']
Vegetables: ['carrot']
Dairy: []
Step-by-Step Explanation:
- Importing defaultdict:
- We import defaultdict from the collections module.
- Creating the defaultdict:
- We pass list as an argument to defaultdict, meaning the default value for missing keys will be an empty list.
- Appending Items:
- We add items to the fruits and vegetables keys using append(). If the key doesn’t exist, it’s automatically created with an empty list as the value.
- Accessing Non-Existent Key:
- Accessing the ‘dairy’ key returns an empty list instead of raising a KeyError.
3. Merging Dictionaries
Python provides several ways to merge dictionaries, depending on the version you’re using. One of the most efficient ways (introduced in Python 3.9) is the union operator (|).
Example:
Let’s merge two dictionaries containing the population of different cities.
# Dictionaries to be merged
city_population_2020 = {
'New York': 8000000,
'Los Angeles': 4000000
}
city_population_2021 = {
'Los Angeles': 4100000, # Updated population
'Chicago': 2700000
}
# Merging the dictionaries using the union operator
merged_population = city_population_2020 | city_population_2021
# Printing the merged dictionary
print(merged_population)
Output:
{'New York': 8000000, 'Los Angeles': 4100000, 'Chicago': 2700000}
Step-by-Step Explanation:
- Initial Dictionaries:
- We have two dictionaries: city_population_2020 and city_population_2021, which contain the populations of cities for different years.
- Merging with the Union Operator:
- The | operator merges the two dictionaries. If a key exists in both dictionaries (like ‘Los Angeles’), the value from the second dictionary (4100000) overrides the value from the first dictionary.
- Result:
- The result is a new dictionary with the updated and merged data.
Dictionary Methods and Functions
1. .get() – Safely Access Values
In Python dictionaries this method is used to access the value associated with a key. If the key doesn’t exist, .get() returns a default value instead of raising an error.
Example:
person = {'name': 'Alice', 'age': 25}
# Using .get() to access a key
age = person.get('age') # Returns 25
# Providing a default value for non-existent key
address = person.get('address', 'Unknown') # Returns 'Unknown'
print(age, address)
Output:
25 Unknown
2. .keys() – Retrieve All Keys
In Python dictionaries this method returns a view object containing all the keys in the dictionary.
Example:
fruits = {'apple': 10, 'banana': 5, 'orange': 8}
# Getting all keys
keys = fruits.keys()
print(keys)
Output:
dict_keys([‘apple’, ‘banana’, ‘orange’])
3. .values() – Retrieve All Values
This method in Python dictionaries returns a view object containing all the values in the dictionary.
Example:
fruits = {'apple': 10, 'banana': 5, 'orange': 8}
# Getting all values
values = fruits.values()
print(values)
Output:
dict_values([10, 5, 8])
4. .items() – Retrieve All Key-Value Pairs
In Python Dictionaries, this method returns a view object that contains all the key-value pairs in the dictionary as tuples.
Example:
fruits = {'apple': 10, 'banana': 5, 'orange': 8}
# Getting all key-value pairs
items = fruits.items()
print(items)
Output:
dict_items([(‘apple’, 10), (‘banana’, 5), (‘orange’, 8)])
5. .update() – Merge or Update Dictionaries
This method updates the dictionary with elements from another dictionary or an iterable of key-value pairs. If the key already exists, its value will be updated.
Example:
fruits = {'apple': 10, 'banana': 5}
new_fruits = {'orange': 8, 'apple': 12}
# Updating the fruits dictionary
fruits.update(new_fruits)
print(fruits)
Output:
{‘apple’: 12, ‘banana’: 5, ‘orange’: 8}
6. .pop() – Remove a Key-Value Pair and Return Its Value
This method removes the specified key and returns its value. If the key doesn’t exist, it raises a KeyError, but you can provide a default return value to avoid the error.
Example:
fruits = {'apple': 10, 'banana': 5}
# Removing 'banana' and getting its value
removed_value = fruits.pop('banana')
print(fruits)
print(removed_value)
Output:
{‘apple’: 10}
5
7. .popitem() – Remove and Return the Last Key-Value Pair
This method removes and returns the last inserted key-value pair as a tuple. If the dictionary is empty, it raises a KeyError.
fruits = {'apple': 10, 'banana': 5, 'orange': 8}
# Removing the last item
last_item = fruits.popitem()
print(fruits)
print(last_item)
Output:
{‘apple’: 10, ‘banana’: 5}
(‘orange’, 8)
8. .clear() – Remove All Items
This method removes all key-value pairs from the dictionary, leaving it empty.
Example:
fruits = {'apple': 10, 'banana': 5, 'orange': 8}
# Clearing the dictionary
fruits.clear()
print(fruits)
Output:
{}
9. .copy() – Create a Shallow Copy
This method returns a shallow copy of the dictionary. Changes to the original dictionary won’t affect the copy, but modifications to nested objects will affect both.
Example:
fruits = {'apple': 10, 'banana': 5}
# Creating a shallow copy
fruits_copy = fruits.copy()
# Modifying the copy
fruits_copy['banana'] = 8
print(fruits)
print(fruits_copy)
Output:
{‘apple’: 10, ‘banana’: 5}
{‘apple’: 10, ‘banana’: 8}
10. .setdefault() – Get Value or Insert Default
This method returns the value of a key if it exists. If not, it inserts the key with a default value.
fruits = {'apple': 10, 'banana': 5}
# Getting an existing key
apple_value = fruits.setdefault('apple', 0) # Returns 10
# Inserting a non-existent key
grape_value = fruits.setdefault('grape', 12) # Adds 'grape': 12
print(fruits)
Output:
{‘apple’: 10, ‘banana’: 5, ‘grape’: 12}
Other Useful Functions with Dictionaries
len() – Get the Number of Items
This function returns the number of key-value pairs in the dictionary.
Example:
fruits = {'apple': 10, 'banana': 5, 'orange': 8}
# Getting the number of items
length = len(fruits)
print(length)
Output: 3
in – Check if Key Exists
The in operator checks whether a specified key exists in the dictionary.
Example:
fruits = {'apple': 10, 'banana': 5}
# Checking if 'apple' exists
exists = 'apple' in fruits
print(exists)
Output: True
Conclusion
These methods and functions make it easy to manipulate and manage dictionaries in Python. Whether you’re accessing values, modifying items, or checking for the existence of keys, these built-in tools will help you handle dictionaries effectively in your programs.