Python, a powerful and versatile programming language, is widely used for web development, data analysis, artificial intelligence, and scientific computing. One of the most fundamental and frequently used data structures in Python is the list. This guide aims to provide an in-depth understanding of Python lists, their functionality, and various operations that can be performed on them.
- What is a Python List?
- Creating Python Lists
- Accessing List Elements
- Slicing Lists
- Modifying Lists
- Adding Elements to a List
- Removing Elements from a List
- List Comprehensions
- Common List Operations
- Nested Lists
- Copying Lists
- List Methods Summary
What is a Python List?
A list in Python is an ordered collection of items that can hold a variety of data types such as numbers, strings, and even other lists. Lists are mutable, meaning their contents can be changed after they are created. They are defined by enclosing elements in square brackets, separated by commas.
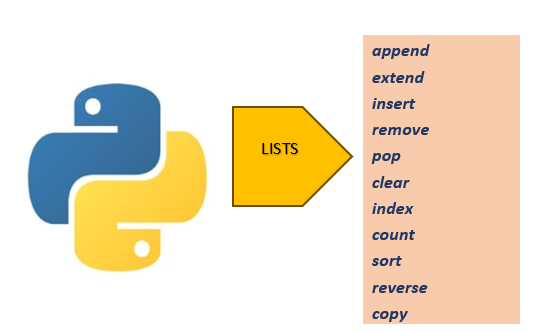
Here is a simple example:
my_list = [1, 2, 3, 'four', 5.0]
Creating Python Lists
Creating a list in Python is straightforward. You can create an empty list or a list with predefined elements.
Empty List
empty_list = []
List with Elements
fruits = ['apple', 'banana', 'cherry']
Accessing List Elements
Each element in a list has an index, starting from 0 for the first element. You can access list elements using their index.
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
print(fruits[2]) # Output: cherry
Negative indices can also be used to access list elements from the end.
print(fruits[-1]) # Output: cherry
print(fruits[-2]) # Output: banana
Slicing Lists
Slicing allows you to access a range of elements in a list. The syntax for slicing is [start:stop:step].
print(fruits[0:2]) # Output: ['apple', 'banana']
print(fruits[1:]) # Output: ['banana', 'cherry']
print(fruits[:2]) # Output: ['apple', 'banana']
print(fruits[::2]) # Output: ['apple', 'cherry']
Modifying Lists
Lists are mutable, meaning you can change their elements. You can use indexing to update individual elements or slicing to update multiple elements.
Updating an Element
fruits[1] = 'blueberry'
print(fruits) # Output: ['apple', 'blueberry', 'cherry']
Updating Multiple Elements
fruits[0:2] = ['pear', 'kiwi']
print(fruits) # Output: ['pear', 'kiwi', 'cherry']
Adding Elements to a List
You can add elements to a list using various methods like append(), extend(), and insert().
Append Method
The append() method adds a single element to the end of the list.
fruits.append('orange')
print(fruits) # Output: ['pear', 'kiwi', 'cherry', 'orange']
Extend Method
The extend() method adds multiple elements to the end of the list.
fruits.extend(['grape', 'melon'])
print(fruits) # Output: ['pear', 'kiwi', 'cherry', 'orange', 'grape', 'melon']
Insert Method
The insert() method adds an element at a specified position.
fruits.insert(1, 'pineapple')
print(fruits) # Output: ['pear', 'pineapple', 'kiwi', 'cherry', 'orange', 'grape', 'melon']
Removing Elements from a List
You can remove elements from a list using methods like remove(), pop(), and clear().
Remove Method
The remove() method removes the first occurrence of a specified value.
fruits.remove('kiwi')
print(fruits) # Output: ['pear', 'pineapple', 'cherry', 'orange', 'grape', 'melon']
Pop Method
The pop() method removes and returns the element at a specified position. If no index is specified, it removes the last element.
fruit = fruits.pop(2)
print(fruit) # Output: cherry
print(fruits) # Output: ['pear', 'pineapple', 'orange', 'grape', 'melon']
Clear Method
The clear() method removes all elements from the list.
fruits.clear()
print(fruits) # Output: []
List Comprehensions
List comprehensions provide a concise way to create lists. The syntax is [expression for item in iterable if condition].
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Common List Operations
Length of a List
The len() function returns the number of elements in a list.
print(len(fruits)) # Output: 6
Check if an Element Exists
The in keyword checks if an element exists in the list.
print('apple' in fruits) # Output: False
Sorting a List
The sort() method sorts the list in ascending order by default.
fruits.sort()
print(fruits) # Output: ['grape', 'melon', 'orange', 'pear', 'pineapple']
Reversing a List
The reverse() method reverses the order of the list.
fruits.reverse()
print(fruits) # Output: ['pineapple', 'pear', 'orange', 'melon', 'grape']
Nested Lists
Lists can contain other lists, which are called nested lists.
nested_list = [[1, 2, 3], ['a', 'b', 'c'], [4.5, 5.5, 6.5]]
print(nested_list[1][2]) # Output: c
Copying Lists
You can copy a list using various methods like slicing, the copy() method, and the list() function.
Using Slicing
new_list = fruits[:]
Using copy() Method
new_list = fruits.copy()
Using list() Function
new_list = list(fruits)
List Methods Summary
- append(): Adds an element to the end of the list.
- extend(): Adds multiple elements to the end of the list.
- insert(): Inserts an element at a specified position.
- remove(): Removes the first occurrence of a specified value.
- pop(): Removes and returns an element at a specified position.
- clear(): Removes all elements from the list.
- index(): Returns the index of the first occurrence of a specified value.
- count(): Returns the number of occurrences of a specified value.
- sort(): Sorts the list in ascending order.
- reverse(): Reverses the order of the list.
- copy(): Returns a shallow copy of the list.
By mastering Python lists, you can significantly enhance your programming skills and tackle complex problems more efficiently. Lists are incredibly versatile and, when used effectively, can make your code more readable and efficient.